React is a front-end Javascript library that is widely adopted for building web applications.
Ethers.js is a web 3.0 library that can be used for interacting with smart contracts on the Ethereum blockchain and other Ethereum Virtual Machine (EVM) compatible blockchains.
In this tutorial, I will cover how to use Ethers.js with React to build a front-end web application that can:
- Connect to a Metamask wallet in the browser
- Read the balance of the wallet
- Access the current block of the Ethereum network
- Listen to updates to the block of the Ethereum network
- Read data from a smart contract
- Make a write transaction to a smart contract via Metamask
Creating a React Project with Create React App
First, we will create a new React project using the create-react-app utility.
If you don’t have the create-react-app utility on your computer, you can download it via npm with the following command.
npm install -g create-react-app
We will create a new React project with the name react-ethersjs with the following command.
npx create-react-app react-ethersjs
Once the project has been created, change the directory into your project and then you can test the app has been successfully created with the command
yarn start
This should display your web application in a new browser tab.
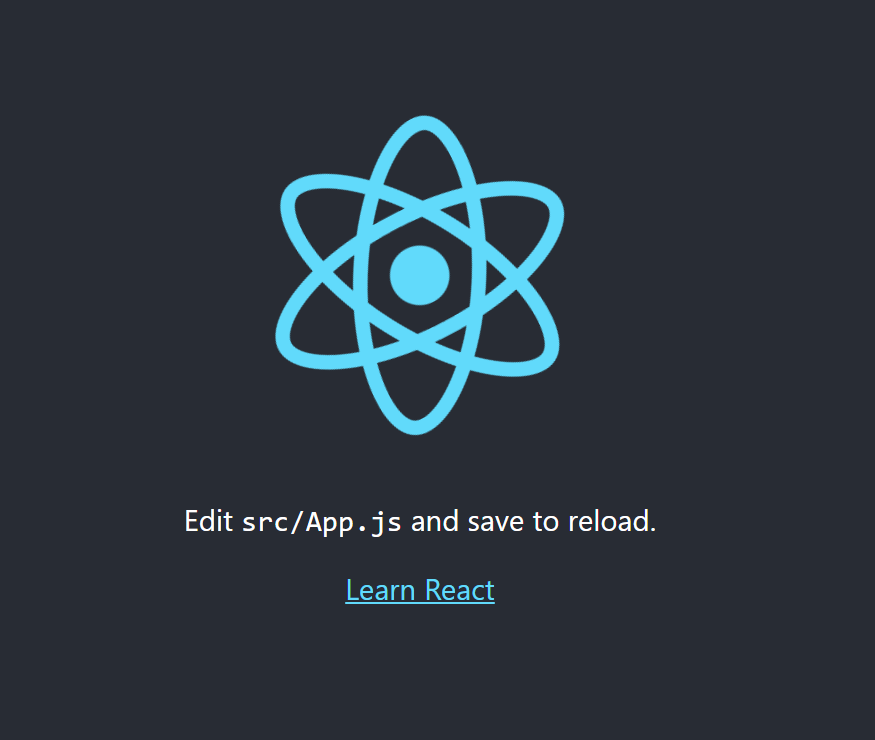
How to Install Ethers.js
Ethers.js is simple to install.
To install using yarn inside the root directory of your React project, run the command
yarn add ethers
Alternatively, you can install Ethers.js with npm with the following command
npm install --save ethers
How to Connect to Metamask using Ethers.js
Metamask is a popular cryptocurrency wallet that is used in browsers as a browser extension or add-on.
It has the ability to interact with decentralized applications (DApps).
In order to connect a Metamask wallet to a React app, we will first create a new React component for connecting Metamask to the React app with Ethers.js.
We will then need to update index.js to replace the reference to App with the Metamask component.
To use the Ethers.js library in your React project, you will need to add the following import statement above your class definition.
import { ethers } from "ethers";
Then we will create a new function that uses the Ethers.js library to connect to a users’ Metamask wallet within the web browser to the React app.
Finally, we will add a button to the user interface with the label “Connect to Metamask”, that we can use to connect to the user’s Metamask wallet by creating a Web3Provider using Ethers.js, then getting the connected account address using the eth_requestAccounts method.
How to Get the ETH Balance of a Metamask Account Using Ethers.js
To get the ETH balance of a Metamask account using Ethers.js, you can use the getBalance function on the Web3Provider passing the wallet address as a parameter.
The function will return a BigNumber, to display this in ETH units in the user interface, use the utility function formatEther.
I have updated the Metamask class created in the previous section to retrieve the users’ Ethereum balance from their Metamask account using Ethers.js and then display the formatted balance in ETH units in the user interface.
How to Get the Current Ethereum Block using Ethers.js
Ethers.js can be used to get the current Ethereum block number using the getBlockNumber function on the Web3Provider.
You can also listen to new updates to the Ethereum block number using the on function on the Web3Provider in Ethers.js
I have updated the Metamask class to display the current block number and listen to changes in the block number to be updated within the user interface.
How to Read Data from Smart Contracts using Ethers.js
Ethers.js can be used to read data from smart contracts.
Before you will be able to do this, you will need two things.
- The smart contract ABI
- The smart contract address
The term ABI stands for Application Binary Interface (ABI). This is a JSON file that describes the functions and events that are available on a smart contract.
For each function, the ABI describes:
- The name of the function
- The names and data types of each of the parameters
- The return value(s) names and data types
- Whether the function is constant
- Whether the function is payable
- The state mutability of the functions
For each event, the ABI describes:
- The name of the event
- The names and data types of each of the parameters
- Whether the event is anonymous
This is an example of an Application Binary Interface (ABI) for an ERC20 token.
In our React application, we will be using the ABI above to load the balance of an ERC20 token residing in the users’ Metamask wallet.
We will use the DAI stable coin ERC20 token in our example. The contract address of DAI on the Ethereum network is 0x6B175474E89094C44Da98b954EedeAC495271d0F.
See the code sample below showing how to access the users’ DAI balance and display it in the user interface.
How to Call Writable Functions on Smart Contracts Using Ethers.js
Ethers.js can also be used within a React application to invoke write functions on a smart contract.
For example, if you want to transfer a certain amount of ERC20 tokens to another wallet address, this can be achieved by calling the transfer function on the smart contract and passing the sender address and the number of token units you want to send.
See the code sample below showing how to initiate a Metamask transaction to send 1 DAI as a donation.